Stop Displaying a Campaign After Conversion
In this tutorial, we will explain how to use the Prisma Campaigns API to stop displaying a campaign to a customer who has converted. The following concepts and steps apply to all cases that use the SDK in an external tool.
Before getting started
The campaign used in this article uses an HTML banner and has a single step of redirection to an internal URL. The application is responsible for 1) managing this action and displaying a given functionality, and 2) continuing the funnel flow to convert.
Campaign configuration
After creating a campaign and enabling the Banners channel, we will add an HTML banner with the content shown below:
<a onclick="prisma.startFunnel.call(this)" href="#">
<span>I'm interested</span>
</a>
To set up the redirection to an internal URL, we will define a funnel step of the corresponding type by checking Open redirect in a new window and leaving Convert when redirection is done unchecked. This decision follows the proposed goal of letting the external tool be in charge of indicating the client’s conversion, starting with the interception of the internal URL (BM://TCRenovacion in the image below):
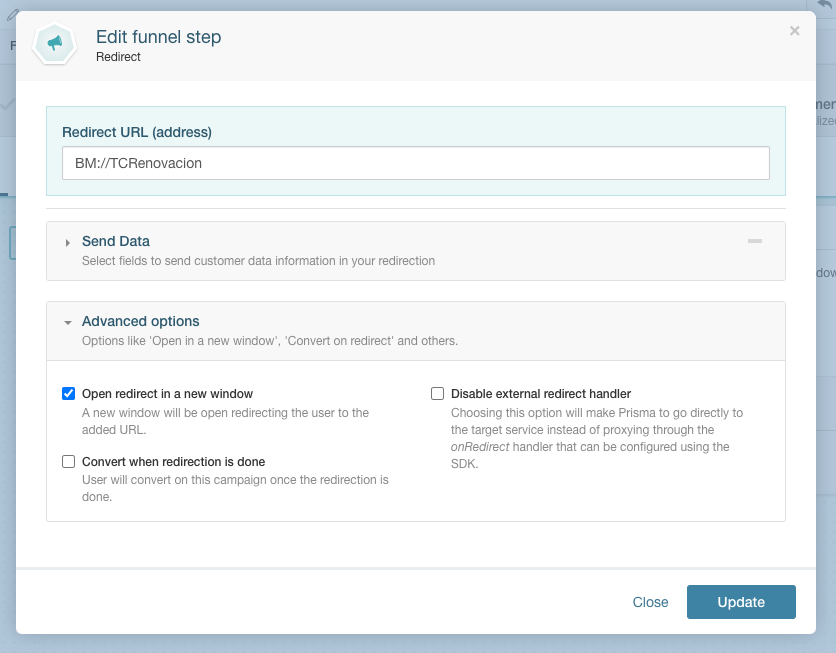
To hide the campaign from the customer after conversion, we will need to check the option Don’t show campaign after customer converts in the configuration:
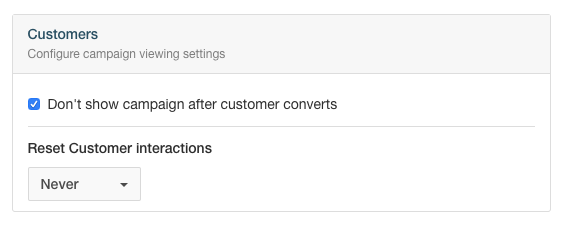
Before proceeding, we must be sure to save the changes and publish the campaign.
Integrating with an external application
To begin with, we will define an OnRedirect
callback to intercept the redirects whose URL starts with BM and execute the function that invokes the desired functionality. In this case, we use loadInternalFunnel
for this purpose:
prisma.load(SERVER,
PORT,
APP_TOKEN,
CUSTOMER_ID,
INTEGRATIONS,
PROTOCOL,
{
onRedirect: function(url, params) {
if (url.startsWith('BM://')) {
loadInternalFunnel(params["trail-id"], params["tracking-token"]);
} else {
window.open(url);
}
}
});
In the current example, we use BM as an internal navigation protocol, but you can use a different one according to the needs of the solution. In case the redirection is external, you can do a regular redirection through
window.open
or some other convenient mechanism.
To manipulate the internal navigation, we will use two functions to load the funnel (loadInternalFunnel
) and to perform the conversion (prismaConvert
) after completing it:
var prismaConvert = function(trailId, trackingToken) {
var convertURL = PROTOCOL + "//" + SERVER + ":" + PORT + "/api/traces/trail/" + trailId + "/action";
const xhr = new XMLHttpRequest();
xhr.onload = () => {
if (xhr.status >= 200 && xhr.status < 300) {
// Add code to handle conversion here
console.log("Converted!");
}
};
const json = {
"name": "conversion",
"type": "conversion",
"data": [
{
"name": "tracking-token",
"value": trackingToken
}
]
};
xhr.open('POST', convertURL);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.send(JSON.stringify(json));
}
var loadInternalFunnel = function(trailId, trackingToken){
prismaConvert(trailId, trackingToken);
}
If the call to the conversion API is successful, three things should happen:
-
The campaign will not be displayed to the customer even if the banners are requested again. When inspecting the network traffic using the browser’s developer tools, you will see a failed request with a 410 error code. This fact indicates that the client traces need to be renewed because the previous ones were closed after conversion.
-
The conversion record will appear in Customer Journey:
-
The statistics in the campaign analysis will show the conversion:
Related Articles
On this page