JavaScript parameters, integrations, and examples (SDK)
Prisma Campaigns provides a library that includes a set of controls to perform the integration using JavaScript. We recommend using this strategy with web applications (both desktop and mobile) and other solutions developed using frameworks with JavaScript support (Phonegap/Cordova and Titanium, to name two examples). Thus, it is not necessary to resort to other external resources to ensure the correct operation of web channels.
Installing the SDK
Before using the library in a web environment, you need to insert the code below inside the <body>
element:
<script type='text/javascript' src='http://SERVER:PORT/sdk/javascript/prisma.js'></script>
<script type = "text/javascript" >
prisma.load(SERVER, PORT, APP_TOKEN, CUSTOMER_ID, INTEGRATIONS, PROTOCOL, OPTIONS);
</script>
The SERVER, PORT, and APP_TOKEN variables are application-specific and are available in Settings/Applications, while CUSTOMER_ID and the INTEGRATIONS vector are the responsibility of the person in charge of the integration. If you select the target channel in this section, you will be able to access the integration code with the preloaded values.
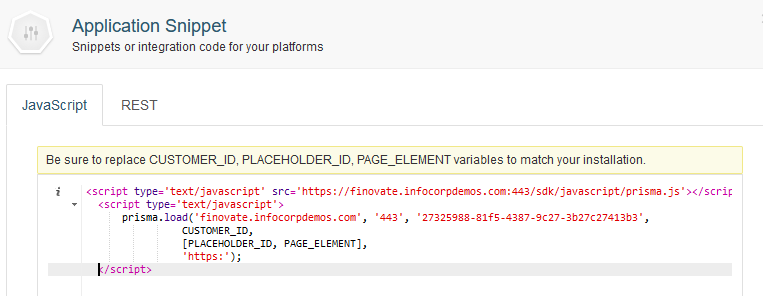
The following table shows more details about each integration parameter:
Parameter | Data type | Required | Description |
---|---|---|---|
SERVER | string | Yes | Server name where Prisma Campaigns is installed |
PORT | string | Yes | Listening port |
APP_TOKEN | string | Yes | Access token for the current application |
CUSTOMER_ID | string | Yes | Customer ID (if available), or an empty string ("") otherwise |
INTEGRATIONS | vector | Yes | Vector with the list of placeholders to be integrated into the current page |
PROTOCOL | string | No | Connection protocol (http or https) based on SSL availability |
OPTIONS | string | No | Optional integration parameters, callbacks, and other elements |
It is important to note that this code will load the library asynchronously, which means the site will load fast despite the dependency.
Integrating Placeholders
This section describes the keys allowed in each element of the INTEGRATIONS vector:
Key | Data type | Required | Description |
---|---|---|---|
placeholderId | string | Yes | Placeholder ID as configured in Prisma Campaigns |
elemendId | string | Yes | HTML element ID where placeholder banners will be loaded |
unrolled | boolean | No | If the placeholder supports multiple campaigns, this parameter indicates if all of them will be displayed simultaneously (unrolled) or not. The default value is false. |
fallbackUrl | string | No | Fallback URL in case the campaign platform is not available |
context | Object | No | Placeholder context that can be used to filter qualifying campaigns |
isolated | boolean | No | This parameter enables displaying a banner in an isolated iframe to avoid affecting the page styles. The default value is true. |
Example 1 - Initializing 3 separate placeholders:
<script type='text/javascript'>
prisma.load('localhost', '443', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [
{placeholderId: "MainHomeBanner", elementId: "banner_flash"},
{placeholderId: "RightHomeBanner", elementId: "banner_right"},
{placeholderId: "LeftHomeBanner", elementId: "banner_left"}
],
"https:");
</script>
Example 2 - Integrating a single placeholder in unrolled carrousel mode with context filter:
<script type='text/javascript'>
prisma.load('localhost', '443', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [
{placeholderId: "PromocionesHome",
context: {"productId" : "1234"},
unrolled : true,
elementId : "promo_list"}
],
"https:");
</script>
Note: The above examples use the 137-W fixed value as customer ID. To implement the integration in a production environment, this field must be populated dynamically for each customer.
Optional Parameters
This section lists the keys allowed in the OPTIONS vector:
Key | Data type | Description |
---|---|---|
onLoaded | function | Callback that is executed once the initialization is finished |
onLoadFailed | function | Callback that is invoked in case there are any errors during the initialization process |
onRedirect | function | Callback that is triggered after a funnel redirection |
onPopup | function | Callback that controls the display of popups |
storageType | string | Determines how browsing information will be stored (local or cookies , where the former is the default) |
reloadPopupsOnReset | boolean | Determines if popups should be shown after reloading the integration (after conversion, for example), with false as the default value |
language | string | Indicates the internationalization language (es by default) |
useTranslator | boolean | Indicates if internationalization will be used (true by default) |
channel | string | Channel being integrated (web by default) |
platform | string | Determines the integration platform with the browser name as default value (chrome , firefox , etc) |
Next, we will illustrate the use of the onLoaded
, onLoadFailed
, and onRedirect
functions.
onLoaded
This function takes as parameters the banners that are available for a given customer:
<div id="container"></div>
<script type='text/javascript'>
var onLoaded = function(banners) {
console.log("Prisma Campaigns was loaded correctly");
};
prisma.load('campaigns.bank.com', '80', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [{"placeholderId": "MainBanner", "elementId": "container"}],
"http:", {onLoaded: onLoaded});
</script>
onLoadFailed
In this case, the optional parameter named error
returns the load error with the following attributes:
Key | Data type | Description |
---|---|---|
data | string | Error information |
status | string | HTTP response code |
<div id="container"></div>
<script type='text/javascript'>
var onLoadFailed = function(error) {
console.log("There was an error during the initialization of Prisma Campaigns");
};
prisma.load('campaigns.bank.com', '80', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [{"placeholderId": "MainBanner", "elementId": "container"}],
"http:", {onLoadFailed: onLoadFailed});
</script>
onRedirect
This function takes as parameter the redirection URL:
<div id="container"></div>
<script type='text/javascript'>
var onRedirect = function(url) {
console.log("You will be redirected to" + url);
};
prisma.load('campaigns.bank.com', '80', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [{"placeholderId": "MainBanner", "elementId": "container"}],
"http:", {onRedirect: onRedirect});
</script>
Examples
This section illustrates a few use cases with a brief detail about their implementation. Although there are no restrictions as to the HTML element where banners will be displayed, it will usually be a <div>
.
Anonymous Public Access
In this scenario, you have a public site with a single placeholder available (Portal - Main Banner) that you want to display in mainbanner and an unidentified customer, so the CUSTOMER_ID parameter is sent empty.
<script type = "text/javascript">
prisma.load("campaigns.bank.com", "80", "175b18e2-08f2-43c2-953a-91f370a0fe23",
"", [{placeholderId : "Portal - Main Banner", elementId : "mainbanner"}]);
</script>
Identified Access
When the person browsing the site is a known client, you should pass the corresponding ID as a parameter to the load
method. The code below assumes that this data is available in session.customer_id
and that the page will show the images inside of the elements identified by left_banner and right_banner:
<script type = "text/javascript">
prisma.load("campaigns.bank.com", "80", "175b18e2-08f2-43c2-953a-91f370a0fe23", session.customer_id,
[{placeholderId : "Position - Left", elementId: "left_banner"},
{placeholderId : "Position - Right", elementId: "right_banner"}]);
</script>
Campaign List
After calling load
as explained previously, you can retrieve all the active campaigns for a specific customer using activeCampaigns
. The syntax of this function and the list of available parameters are as follows:
activeCampaigns(customerId, category, group, fnResults)
Parameter | Data type | Required | Description |
---|---|---|---|
customerId | string | Yes | Customer ID (if available) or an empty string ("") otherwise |
category | string | No | Optional parameter to filter campaigns by category |
group | string | No | Optional parameter to filter campaigns by group |
fnResults | function | No | Asynchronous function that is called upon receiving the response |
The fnResults
function receives a list of campaigns with the following fields: name
, category
, group
, customOffer
and funnelUrl
. By using this resource, you can fetch the names of those campaigns to name an example:
prisma.client.activeCampaigns("137-W", "Sales","Mortgages",
function (campaigns) {
for (var i = 0 ; i < campaigns.length; i++) {
console.log(campaigns[i].name);
}
});
Likewise, you can trigger the conversion funnel of a given campaign in a new window:
prisma.client.activeCampaigns("137-W", "Sales","Mortgages",
function (campaigns) {
for (var i = 0 ; i < campaigns.length; i++) {
if (campaigns[i].customOffer.mortgageRate != undefined) {
campaigns[i].startFunnel();
break;
}
}
});
The above example will retrieve campaigns associated with mortgage sales and will trigger the funnel of the one with higher priority where the mortgageRate
field is defined.
Personalized Carrousel
Through onLoaded
, you can create banners using controls o custom components other than the ones provided in the SDK. To accomplish this goal, you need to take the following items into account:
-
The
elementId
parameter, which represents an HTML element, must benull
. -
The
onLoaded
function takes the list of banners in a dictionary where each key is the placeholder ID.
It is important to note that each item in the response contains a list of images in the images
attribute, in case two or more matching campaigns are found. The next steps consists of displaying the banner or image somewhere in the page using the show
method:
<div id="my-parent"></div>
<script type='text/javascript'>
var onLoaded = function(banners) {
var carouselParent = document.getElementById("my-parent");
var prisma = this;
var banner = banners["MainBanner"];
for (var i = 0; i < banner.images.length; i++) {
var image = banner.images[i];
image.show(carouselParent);
}
};
prisma.load('campaigns.bank.com', '80', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [{"placeholderId" : "MainBanner", "elementId" : null}],
"http:", {onLoaded : onLoaded});
</script>
In this example, the page will display the image or HTML banners associated with MainBanner inside of my-parent.
Personalized Popups
The onPopup
function handles how the application will display popups. To that effect, you need to pass the popup as parameter as onPopup(popup)
:
<div id="my-parent"></div>
<script type='text/javascript'>
var onPopup = function(popup) {
if (popup.banner.category == "Ventas") {
// 1. Display the popup in a personalized manner
// 2. Returning true means that the system should not display the popup since it was done separately
return true;
}
};
prisma.load('campaigns.bank.com', '80', '8e2626da-4ee1-45c7-b269-50df530dbc6e',
"137-W", [{"placeholderId" : "MainBanner", "elementId" : null},],
"http:", {onPopup : onPopup});
</script>
In this case, you should include a callback called onPopup
in the OPTIONS parameter of the load
method, as illustrated above. The integration will invoke the function whenever there is a popup to display among the list of campaigns for the current user.
The popup parameters should be specified as follows:
Parameter | Data type | Required | Description |
---|---|---|---|
width | long | Yes | Placeholder width |
height | string | Yes | Placeholder height |
banner | Banner | Yes | Configuration of the banner to display in the popup |
hideClose | boolean | Yes | Determines if the close button should be hidden, based on the platform configuration |
dismissFn | function | No | Function to execute when the customer dismisses a campaign if this action is allowed |
The banner
parameter has different attributes depending on whether it consists of an image or HTML code. Thus, it allows you to display the popup in a customized way according to the requirements in one of the following ways:
BannerHTML()
Parameter | Data type | Description |
---|---|---|
width | long | Placeholder width |
height | string | Placeholder height |
category | string | Category of the campaign associated with the banner (if available) or an empty string otherwise |
group | string | Group of the campaign associated with the banner (if available) or an empty string otherwise |
Funnel | funnel | Campaign funnel configuration |
isolated | boolean | Indicates if the popup was requested in isolated mode |
content | string | HTML content of the banner |
BannerImage()
Parameter | Data type | Description |
---|---|---|
width | long | Placeholder width |
height | string | Placeholder height |
category | string | Category of the campaign associated with the banner (if available) or an empty string otherwise |
group | string | Group of the campaign associated with the banner (if available) or an empty string otherwise |
Funnel | funnel | Campaign funnel configuration |
link | string | Link of the image to be displayed in the banner |
Conversion
When a customer converts in a given campaign, you can call the convert
method as follows:
<script type='text/javascript' src='http://SERVER:PORT/sdk/javascript/prisma.js'></script>
<script type = "text/javascript" >
prisma.convert(SERVER, PORT, APP_TOKEN, PROTOCOL, CAMPAIGN_ID, CALLBACK)
</script>
The SERVER, PORT, APP_TOKEN and PROTOCOL parameters are the same as in previous cases. The other two are outlined in the table below:
Parameter | Data type | Required | Description |
---|---|---|---|
CAMPAIGN_ID | string | Yes | Campaign ID as given in the redirect URL |
CALLBACK | function | No | The function to execute after conversion takes the API response as parameter |
Usually, a funnel step will redirect to an external tool (web application). In this action, you need to send three parameters within the query (campaign-id, trail-id, and funnel-node-id) that can be obtained in the backend or using JavaScript in the browser:
<script type = "text/javascript" >
function getParameterByName(name, url) {
if (!url) url = window.location.href;
name = name.replace(/[\[\]]/g, "\\$&");
var regex = new RegExp("[?&]" + name + "(=([^&#]*)|&|#|$)"),
results = regex.exec(url);
if (!results) return null;
if (!results[2]) return '';
return decodeURIComponent(results[2].replace(/\+/g, " "));
}
</script>
Cordova
To display HTML banners in a Cordova app, you need to meet one of the following conditions:
- Set
isolated = false
for the placeholder to avoid creating the banner inside of an *iframe. - Add the rule
<allow-navigation href="about:" />
or<allow-navigation href="*" />
to the Cordova config.xml to display the banner iframe.
Related Articles
On this page