iOS
Prisma Campaigns provides a framework library with a set of controls and classes for the integration with iOS using Swift 4. The Library Reference section below explains how to reference the library in your Xcode project in more detail.
First steps
The Prisma client library requires the following initialization parameters:
load(String server, String port, String appToken, String customer, String protocol)
where
param String server
andparam String port
: server name and port where the Prisma service resides and is listening.param String appToken
: access token for the current application.param String customer
: customer ID (if available). Otherwise, pass an empty string ("").param String protocol
: Connection protocol (https by default).
To begin, import the Prisma SDK and invoke the Client.load()
static method only once, preferably in the AppDelegate
class when the application finishes loading:
import Prisma
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
Client.load(server: "localhost", port: "8080", appToken: "22dae0ad-a6a8-4767-80bc-b29098147813", customer: "1234")
return true
}
Since the Client
class is a singleton, it will return the same instance of the object whenever the application calls it through the shared
static attribute as Client.shared!
.
Embedded banners
To integrate a banner into an iOS View and load dynamic content, you can use the Placeholder
control as follows:
First, add a Horizontal Stack View control to the view:
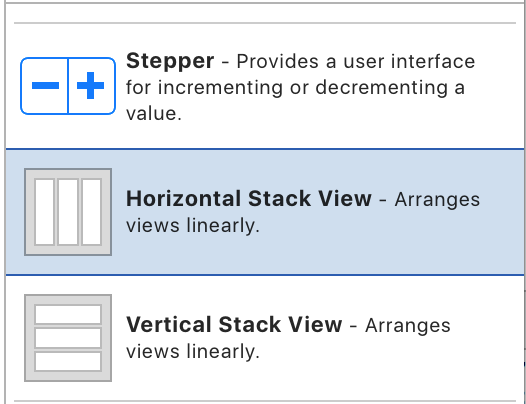
Next, link the control to the Placeholder
class provided by the Prisma framework:
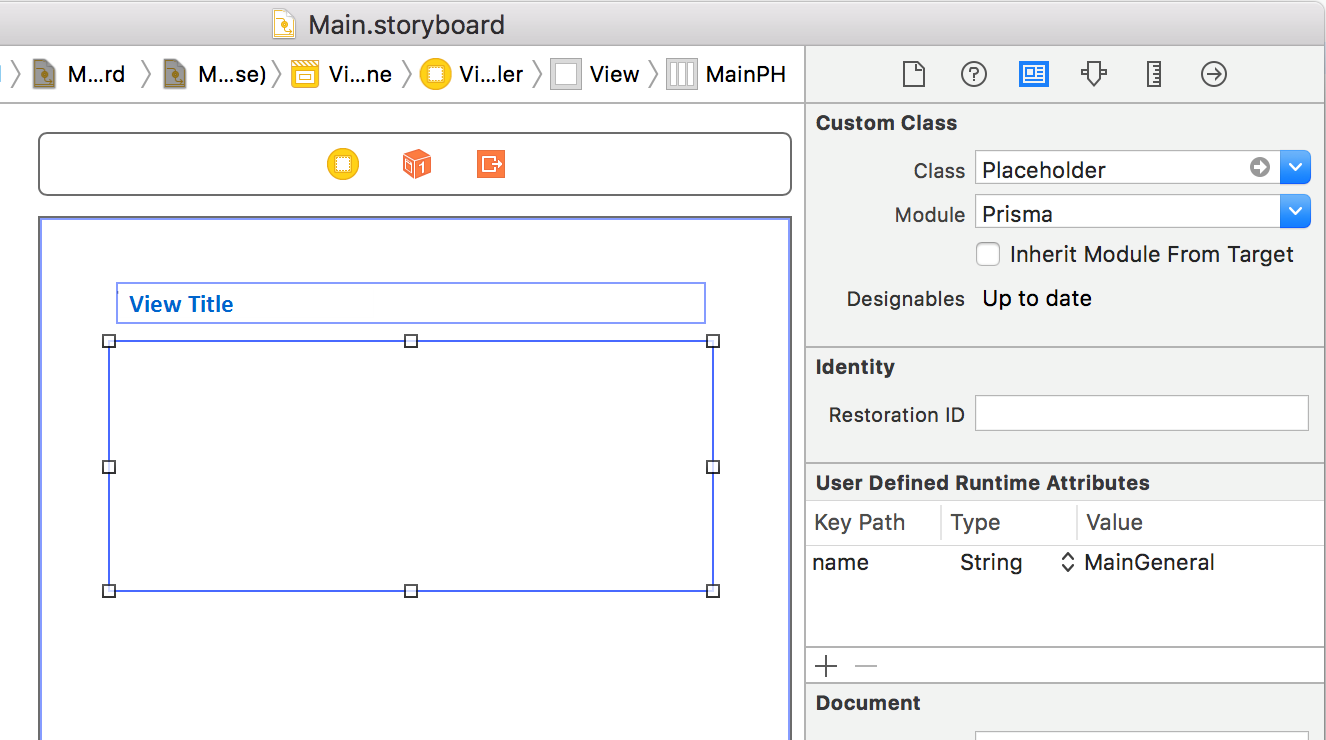
and name the placeholder according to the campaign settings:
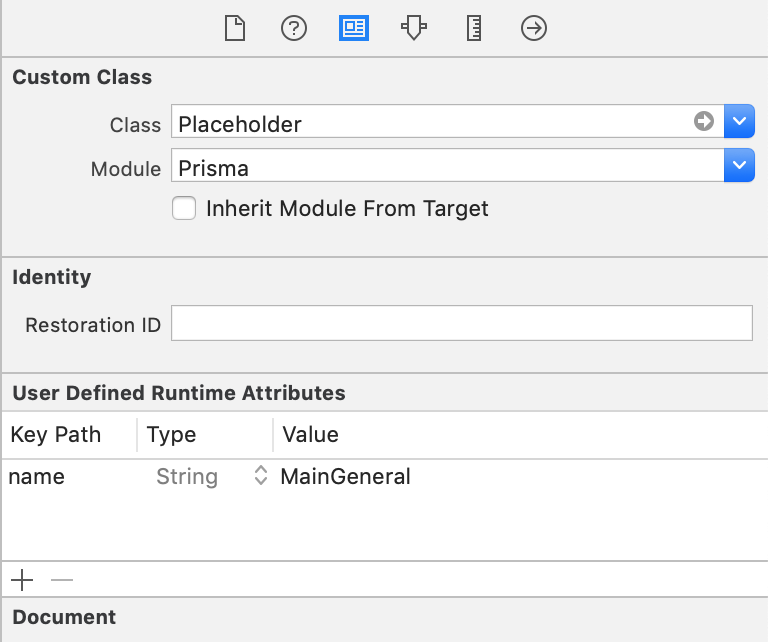
Then create an Outlet for Placeholder
:
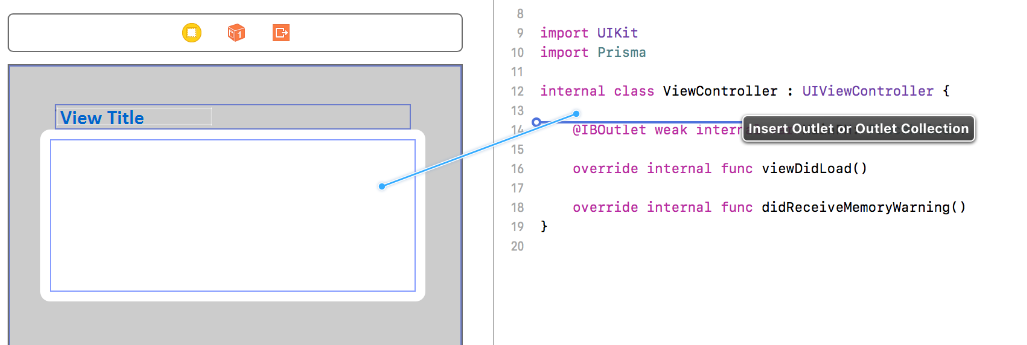
It is worth noting that you should name this variable to reference the placeholder during page synchronization properly.
Finally, synchronize campaigns when creating the view:
import Prisma
class ViewController: UIViewController {
@IBOutlet weak var MainPH: Placeholder!
override func viewDidLoad() {
super.viewDidLoad()
Client.shared!.syncView(viewName: "Main", placeholders: [MainPH])
}
In this case, the placeholders
parameter should include the list of placeholders defined as outlets, whereas viewName
and MainPH
represent the view and outlet names.
Custom banners
You can also create banners from the application code independently of the SDK. To do this, you can utilize the onSynced
parameter of the syncView
method. Under this scenario, the OnSynced
callback is invoked when requesting the banners for the sync activity:
Client.shared!.syncView(viewName: "ViewControllerName", placeholders: [MainPH], onSynced: {(result: Dictionary<String, PlaceholderContent>) -> Void in
if result["MainGeneral"] != nil {
let content:PlaceholderContent = result["MainGeneral"]!
let size = content.banners.count
print("Total banners \(size) found for placeholder")
}
})
To retrieve the views from the content of placeholder:
let banner = content.banners[0]
let v:UIView = banner.getView()
or the content of an HTML banner:
let htmlBanner = banner as! HtmlBannerView
let htmlContent:String = htmlBanner.getHTMLContent()
To initialize the funnel from a banner:
let funnel = banner.getFunnel()
funnel.show()
or dismiss it:
let funnel = banner.getFunnel()
funnel.dismiss()
Dismiss and redirect protocols
Prisma Campaigns exposes extensibility points through the following protocols:
-
PrismaRedirectHandler.onRedirect
is called when a funnel redirect step is executed. For example, you can use this mechanism to browse to an URL viaSFSafariViewController
to prevent the user from exiting the app. By returningtrue
, you can tell Prisma to not execute its default action (going to the URL in Safari). -
PrismaDismissHandler.onDismissed
is invoked when someone dismisses a campaign from a banner, and returnstrue
to indicate that Prisma should not sync the view.
import Prisma
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate, PrismaRedirectHandler, PrismaDismissHandler {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
Client.load(server: "localhost", port: "8080", appToken: "22dae0ad-a6a8-4767-80bc-b29098147813", customer: "1234", redirectHandler: self, dismissHandler: self)
return true
}
func onRedirect(url: URL) -> Bool {
return true;
}
func onDismissed() -> Bool {
return true;
}
}
Library reference
There are two available methods to integrate the Prisma framework into your project:
- Manual integration requires copying
Prisma.framework
into the folder of your project, and then adding the reference as follows:
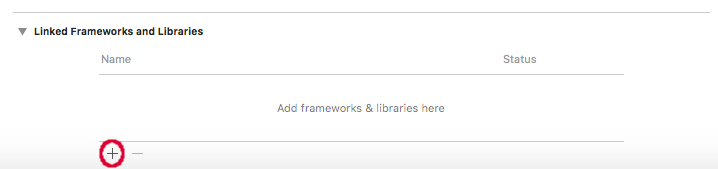
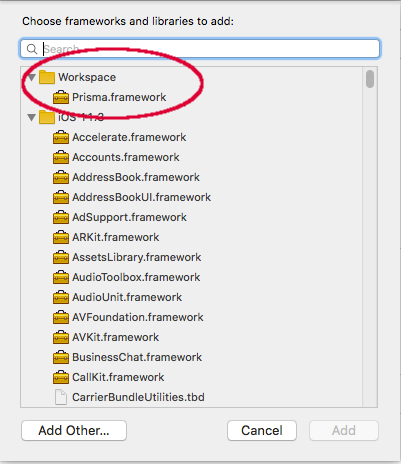
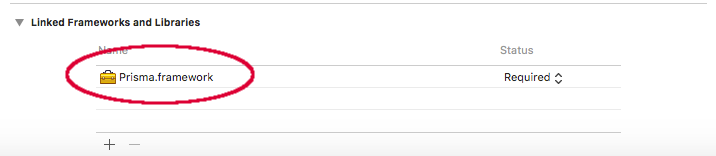
- Integration via CocoaPods involves adding the
Prisma
pod to your Podfile as shown below. Optionally, you can do the same withJumio
:
# Prisma requires iOS 9.0; Jumio requires iOS 10.0
platform :ios, '9.0'
target 'MyProject' do
pod 'Prisma', :podspec => 'https://s3.sa-east-1.amazonaws.com/prisma.sdk/Prisma_Swift5_1.2.2.podspec'
# pod 'JumioMobileSDK/Netverify', '2.14.0'
end
Finally, install the pod to your project with pod install
.
Connectivity permissions
If you need to use http, add the following exception section to the Info.plist
file in your project to explicitly allow insecure connections. To do so, edit the file and replace your-server-address with the IP address or server name of the Prisma Campaigns server you will be using.
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
<key>NSExceptionDomains</key>
<dict>
<key>your-server-address</key>
<dict>
<key>NSExceptionAllowsInsecureHTTPLoads</key>
<true/>
<key>NSIncludesSubdomains</key>
<true/>
</dict>
</dict>
</dict>
On this page