Appcelerator
The Appcelerator Titanium SDK allows developing native, cross-platform mobile applications. Prisma Campaigns supports both the Classic approach and the Alloy (MVC) framework once you copy the corresponding JavaScript components to a project’s directory.
SDK
The SDK consists of 4 files, with prisma.js
being the main one for your integration. Assuming that prisma-sdk -which contains the complete SDK- exists in the current folder, you can import the module as follows:
Prisma = require(prisma-sdk/prisma)
The next step consists of performing the integration itself based on the framework version. However, it is worth noting that the SDK utilizes callbacks -regardless of the version- to load both banners and popups.
Classic approach
To integrate the SDK in Classic mode, copy prisma-sdk as a subdirectory in the Resources folder:
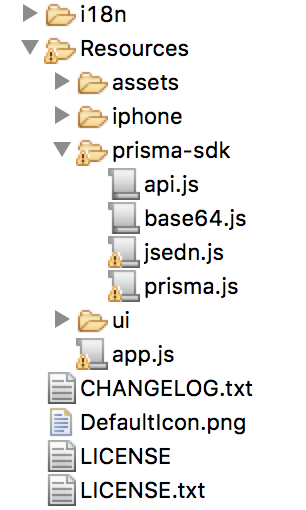
The following example illustrates the strategy where FirstView
integrates a placeholder called PHNAME
, which is synchronized when the view is created:
var Prisma = require('prisma/prisma');
function onBannersLoaded(view, banners)
//banners is a dictionary with key the name of
//each requested placeholder containing the list of images or htmls
//a to display
}
function onPopupFound (view, popup) {
//el popup has the width, hight and banner to display in the following attributes:
//popup.width
//popup.height
//popup.banner
}
function FirstView () {
var self = Ti.UI.createView();
Prisma.load(server, port, app-token, customer-id, [{placeholderId: PHNAME}], protocol,
{onLoaded: function (banners)
onBannersLoaded (self, banners);
},
onPopup: function (popup) {
onPopupFound (self, popup);
}});
return self;
}
module.exports = FirstView;
where the onBannersLoaded
function takes a single image from the list of banners that are available for the given placeholder:
function onBannersLoaded (view, banners)
var placeholderDefinition = banners["MainGeneral"];
// Select the first one banner, if there is more than one a carrousel could be set.
var firstBanner = placeholderDefinition.images[0];
//If the banner has an image link
if (typeof firstBanner.link != undefined)
var image = Ti.UI.createImageView({image: firstBanner.link});
image.addEventListener('click', function(e){image.addEventListener('click', function(e){
Ti.API.info ("Starting funnel");
var url = firstBanner.funnel.getFunnelLanding();
Ti.Platform.openURL (URL);
});
view.add (image);
}
//if the banner has HTML content
else if (typeof firstBanner.content != undefined)
var webview = Ti.UI.createWebView ({
html: firstBanner.content
});
view.add (webview);
}
}
As you can see above, only the first banner is obtained as images[0]
. If more than one banner exists, you can set up a carousel or banner list.
Alloy framework
In this case, you will have to copy the prisma-sdk directory into assets:
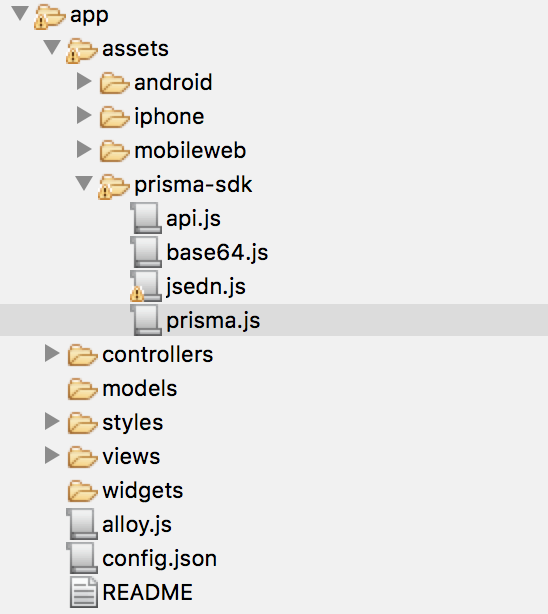
Next, you can integrate it into popups or views with placeholders very easily:
Prisma = require("prisma-sdk/prisma");
function onBannersLoaded (view, banners)
//banners is a dictionary where the keys are the names of
//each requested placeholder containing the list of images or html code
//to display
}
function onPopupFound (view, popup)
// popup contains width, height and the banner to be displayed in the following attributes
//popup.width
//popup.height
//popup.banner
}
function prismaInit(
var self = $.getView();
Prisma.load(server, port, app-token, customer-id, [{placeholderId: PHNAME}], protocol,
{onLoaded: function (banners)
onBannersLoaded (self, banners);
},
onPopup: function (popup)
onPopupFound (self, popup);
}});
return self;
}
$.index.addEventListener('open', prismaInit);
$.index.open();
Under this scenario, the view’s open
event will initialize the campaigns and sync the placeholders by name (as shown by the use of PHNAME
above).
On this page